Java – Convert Array to List
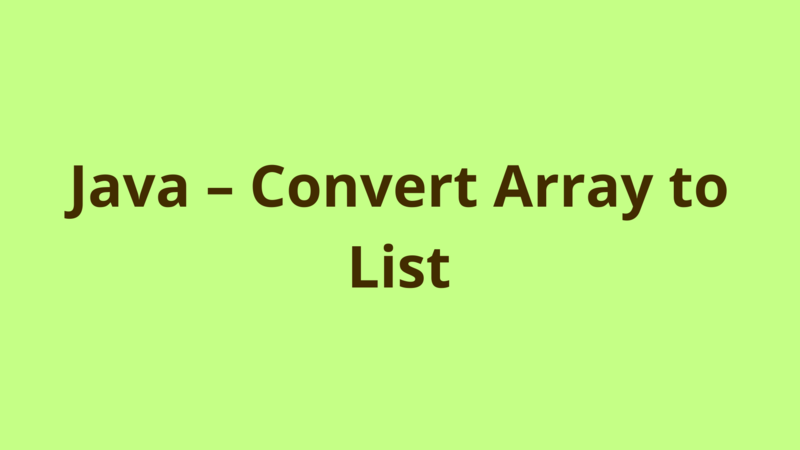
ADVERTISEMENT
Table of Contents
- Introduction
- 1- Arrays.asList
- 1.1- Generate a modifiable list using Arrays.asList()
- 2- Java 8
- 3- Traditional way
- Summary
- Next Steps
Introduction
This tutorial shows several ways to convert Array to List in Java.
1- Arrays.asList
You can convert an Array to List using Arrays.asList() utility method. This method accepts an array as input and returns a list as output.
public static List<String> convertArrayToListAsList(String[] names)
{
List<String> namesLst = Arrays.asList(names);
return namesLst;
}
The limitation of using this method is that it returns a fixed size list.
You can just read and overwrite its elements, however if you try to add/remove elements from the returned list you get UnsupportedOperationException.
It’s worth to mention that modifying the elements of the list affects the original array.
In short, this method should only be used for generating a read-only list.
1.1- Generate a modifiable list using Arrays.asList()
In order to support adding/removing elements from the generated list when using Arrays.asList(), you can initialize a completely independent list out of its result as the following:
List<String> namesLst = new ArrayList<String>(Arrays.asList(names));
2- Java 8
With Java 8, you can convert an Array to List in one line using Arrays.stream() and Collectors.toList() utility methods.
public static List<String> convertArrayToListJava8(String[] names)
{
List<String> namesLst = Arrays.stream(names).collect(Collectors.toList());
return namesLst;
}
Arrays.stream() converts the array into a stream and the stream is then collected as a list using Collectors.toList().
The default type of returned list is ArrayList, in order to decide what type of list to generate use:
Collectors.toCollection(LinkedList::new)
3- Traditional way
You can also do the conversion manually through iterating over the elements of the Array and filling up an ArrayList.
private static List<String> convertArrayToListManually(String[] names)
{
List<String> namesLst = new ArrayList<String>();
for(String name : names)
{
namesLst.add(name);
}
return namesLst;
}
Summary
This tutorial shows several ways to convert Array to List in Java.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers