Python next() Function with Examples | Initial Commit
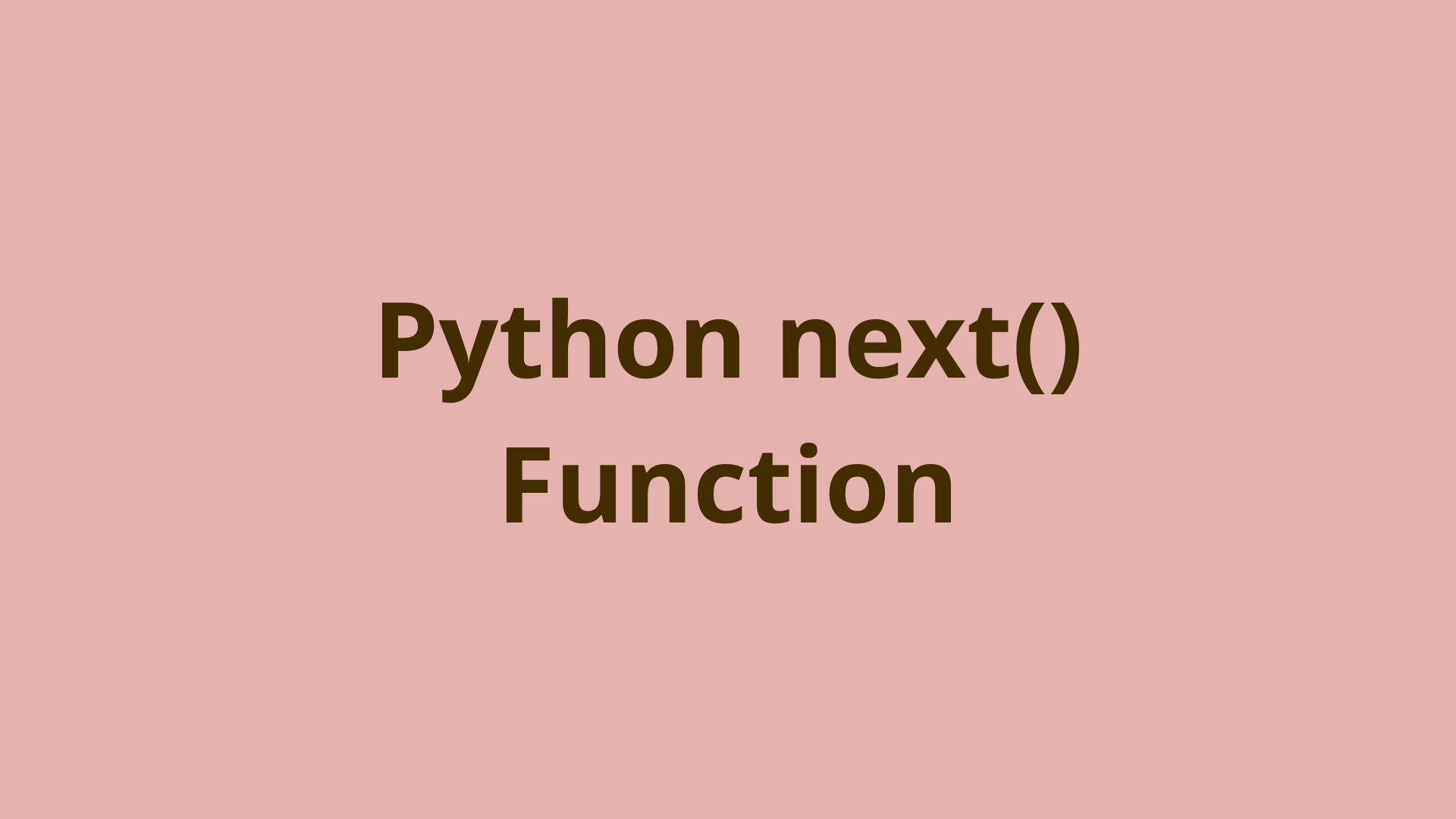
ADVERTISEMENT
Table of Contents
- Introduction
- What is an Iterator in Python?
- What does next() do in Python?
- Python next() - stop iteration with default
- next() with generators
- Summary
- Next Steps
- References
Introduction
Python is a powerful programming language with a wide array of built-in functions to help you accomplish various tasks easily. One such function is the next()
function. It is used on iterators in Python (which we'll discuss later), specifically to get items from those iterators.
You likely have used the next() function in Python without even knowing it since it is used behind the scenes in For loops, to iterate through items.
In this tutorial, you will learn what iterators are, how you can use the next() function on iterators, and maximize your usage of the next() function.
What is an Iterator in Python?
To understand what the next() function is you first have to understand what iterators are in Python.
An iterator is a general python object which contains a collection of objects in order like a tuple or list. However, unlike a list or a tuple, you cannot view these objects all at once. Instead, you have to get the objects from an iterator one by one in order using the next() or __next__() function.
An iterable much like an iterator is an object having a structure that can be stepped through one element at a time. This is because an iterator is an iterable, but not all iterables are iterators.
For example, Strings, lists, and tuples are all iterables, but not iterators. Both iterables and iterators can also be iterated through by using a For loop.
However, the key difference between an iterable and an iterator is that only an iterator has the special "__next__()" method.
You can turn an iterable into an iterator using either the __iter__()
special method or the iter()
function. For example:
>>> testList = [1, 2, 3]
>>> testList.__iter__()
<list_iterator at 0x7ffa2d2a9340>
>>> iter(l)
<list_iterator at 0x7ffa2d2a9c10>
Here, you can see that both return an iterator for the list testList
.
The __iter__() special method is the internal method Python invokes when iter() is called. In addition, iter() has a second argument sentinel to represent the end of a sequence.
What does next() do in Python?
The next()
function is used to get the next item in the iterator. The syntax for the next() function is:
next(iterator, default)
Here, the argument named iterator
is the iterator, and default
is an optional value discussed later. Let's look at an example:
>>> testList = [1, 2, 3]
>>> iter_testList = iter(testList)
>>> next(iter_testList)
1
>>> next(iter_testList)
2
>>> next(iter_testList)
3
>>> next(iter_testList)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
StopIteration
Here, we again have the list testList
, and we store the iterator of that list in the iter_testList
variable. Then, you can call the next() function to get the next number in the iterator one by one until there are no items and you get the StopIteration
Exception.
Like the __iter__() special method __next__ is also a special method and it is the internal method Python invokes when next() is called. But, the next() function also includes the default
argument.
Python next() - stop iteration with default
In the previous example, when the iterator ran out of data, you got the StopIteration Exception. With the default
argument for the next() function, you can choose what you want to output instead of the StopIteration Exception. For example:
>>> testList = [1, 2, 3]
>>> iter_testList = iter(testList)
>>> next(iter_testList, "No more data!")
1
>>> next(iter_testList, "No more data!")
2
>>> next(iter_testList, "No more data!")
3
>>> next(iter_testList, "No more data!")
'No more data!'
>>> next(iter_testList, "No more data!")
'No more data!'
Here, if you set the default
argument to "No more data!", the string is returned instead of an Exception. This can be really useful when don't want your program to terminate because of an empty iterator, and you get control over what to output in that situation.
next() with generators
You can also use the next() function with a generator the same way you do with an iterator.
A generator is a specific type of iterator. It can be a "one-line for loop" or a function that uses the "yield" statement instead of "return".
For example:
>>> def ex_gen():
... yield 1
... yield 2
... yield 3
...
>>> g = ex_gen()
>>> g
<generator object ex_gen at 0x7f99d1af4ac0>
>>> next(g)
1
>>> next(g)
2
>>> next(g)
3
Here, ex_gen
is a generator function, and by calling ex_gen you get a generator object. You can then use the next() function with the generator and it behaves the same as an iterator.
Summary
In this course, you learned what an iterator is and how you can use the next() function on an iterator.
You started by learning what iterators are and the difference between an iterator and an iterable. Next you learned how to turn an iterable into an iterator using the iter() function or __iter__() method.
Then, you learned how to use the next() function on an iterator and understood the difference between the next() function and __next()__ method.
Following that you learned how you can replace the StopIteration exception output with an output of your own using the default
argument for next(). Finally, you briefly learned what generators are and that you can use the next() function with generators.
Next Steps
If you're interested in learning more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
We hope you enjoyed this post! Feel free to shoot me an email at jacob@initialcommit.io with any questions or comments.
References
- Renaming iterator.next() to iterator.__next__() - https://peps.python.org/pep-3114/
- Python Reference, next - https://python-reference.readthedocs.io/en/latest/docs/functions/next.html
Final Notes
Recommended product: Coding Essentials Guidebook for Developers